Shader Resource
Overview
Spark provides many powerful capabilities for the creation of Visually Rich applications - with animations, image masking, clipping and other technologies. The addition the Shader Resource provides near limitless visual effects capabilities - allowing OpenGL shader (programable pipeline) power to be leveraged in Spark applications.
The Shader Resource will allow application developers to harness GPU performance to provide "next-level" visuals.
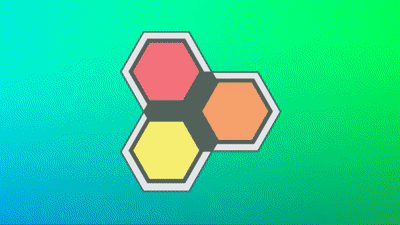
Shader Resource
Spark now supports OpenGL Shaders via the shaderResource. Shaders are written in GLSL - GL Shader language.
Creating the Shader Resource
The following example shows how to create a Shader Resource -
We pass the Shader Resource frag_src and vert_src which are GLSL either URL's to source code, or Data URL's.
Additionally, we declare uniforms that we will use.
In this case,
s_texture
an image bound tou_time
a floating-point time valueu_color
an RGBA color value value.
Using the Shader Resource
The Shader Resource will return a promise to indicate when it's ready. The promise will resolve when the shader code is downloaded and compiled successfullly by OpenGL.
In the case of an error, the promise will reject, and additional details will be available through loadStatus.glError
.
When it's ready, the Shader Resource is attached to the .effect
property of our Image object image
.
It can be attached to any Spark object:- object, rectangle, image
etc.
The configuration for our Shader Resource is assigned as a JSON object as shown above.
We configure uniform values here also.
Shader Configuration
3 methods of configuration are available.
- Direct Uniform Configuration
- Configuration Object - SINGLE
- Configuration Objects - ARRAY
Direct Uniform Configuration
Using the uniform variable name directly on the Shader Resource object.
NOTE: Direct assignment uniforms should be considered volatile.
For example:-
Configuration Object - SINGLE
For a Single shader pass - use a SINGLE JSON configuration object with the 'shader'
Shader Resource object and 'uniforms'
to the Spark object via .effect
.
For example:-
Configuration Objects - ARRAY
For Multiple shader passes - use an ARRAY of JSON configuration objects with the 'shader'
Shader Resource object and 'uniforms'
to the Spark object via .effect
.
For example:-
Inline Source Code
Using Data URL's - the shader source code can be embedded in the Javascript source - and remove the need for extra files / downloads.
NOTE: The use of back ticks >> ` code `
<< in JS code to capture source code as a string.
There is quite a bit going on above.
The code creates a Javascript string as a Data URL capturing fragment shader code which blends source pixels with a color assigned by the uniform u_color
Internal Variables
NOTE: The following GLSL variables are provided by Spark - their names are reserved and should be avoided.
The texture of the Spark object upon which the shader is applied.u_texture
u_resolution
u_time
The resolution / dimensions of the object upon which the shader is applied.
A monotonic increasing, floating-point clock value.
Flattened Composition - u_texture
The Shader Resource will provide a texture of flattened/compositied of the Object and its Children to which shader is applied.
In this case the blue background rounded rectangle
or image
, text and logo image
are rendered to a texture bound to u_texture
.
This texture is accessed in Shader code via u_texture
and may be used in fragment operations.
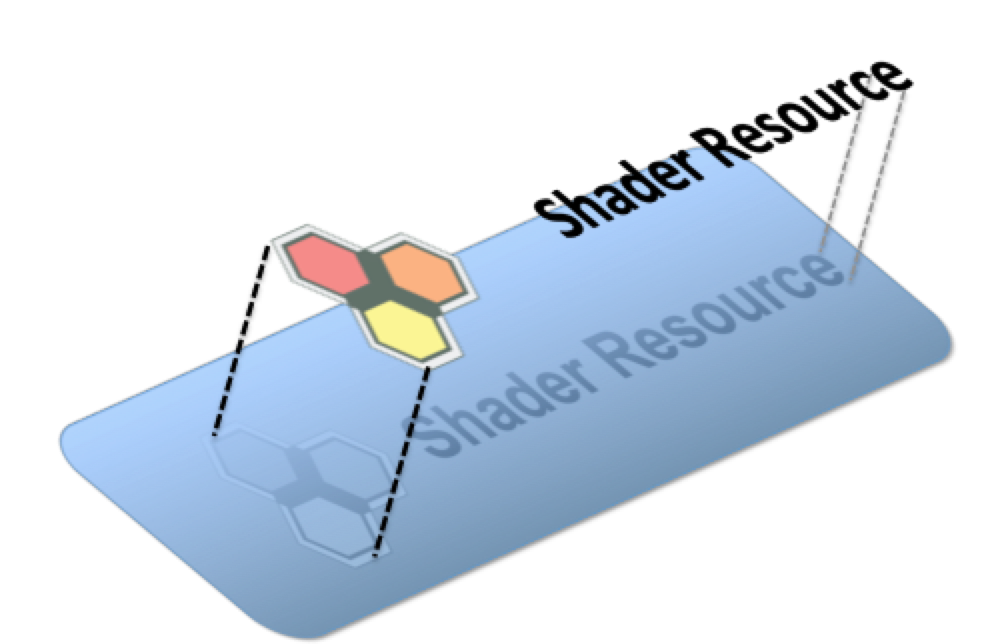
The resulting texture u_texture
can use in fragment sampling operations.
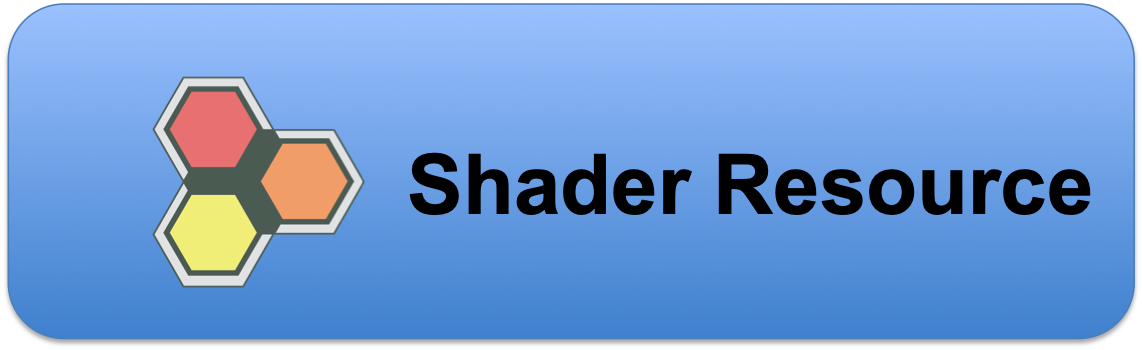
Capability
The following code shows how to test the supported capability of Shader Resource in Spark releases.
Versions
The following code shows how to check the version of Shader Resource in Spark releases.
Further Reading
A great resource for Shader inspiration is Shadertoy.com
It's an open-source gallery of MANY many Fragment Shaders.
Examples
Samples from Shadertoy.com running in Spark ...
It's an open-source gallery of MANY many Fragment Shaders.
Additional Examples
Additional Spark examples can be found here >>> Spark examples